how to have java code fill a form-fillable pdf
How to Programmatically Fill PDF Forms in Java
This guide details how Java code can populate fillable PDF forms. Leverage libraries like Apache PDFBox, iText, or Aspose.PDF for Java to achieve this. Methods involve accessing fields, setting values, and handling various field types.
Choosing a Java PDF Library
Selecting the right Java PDF library is crucial for efficient form filling. Popular choices include Apache PDFBox (open-source, versatile, and widely used), iText (commercial, powerful features, excellent support), Aspose.PDF for Java (commercial, known for ease of use and comprehensive functionality), and Spire.PDF for Java (another commercial option with a strong focus on ease of integration). Consider factors like licensing costs, feature sets (support for various form field types, FDF handling), performance, and community support when making your decision. The best library will depend on your project’s specific requirements and budget. Evaluate the documentation and available examples to determine which aligns best with your coding style and project needs.
Accessing and Manipulating Form Fields
Once you’ve chosen a Java PDF library, accessing and manipulating form fields is straightforward. Most libraries provide methods to retrieve fields by name or index. You’ll typically work with objects representing individual fields (text fields, checkboxes, radio buttons, etc.). These objects usually have methods to get and set field values. For example, a text field object might have a setValue
method to input text. For checkboxes or radio buttons, you would use methods to set their selected state. Remember to handle different field types appropriately; each type will have specific properties and methods for manipulation. Efficiently navigating and interacting with fields is key to automating the form-filling process.
Setting Field Values and Refreshing Appearance
After accessing the desired form fields, setting their values is typically done via a library-specific method, often named something like setValue
. This method takes the new value as an argument. However, simply changing the value might not immediately update the visual representation of the form within the PDF. Many libraries require a call to a separate method, such as refreshAppearance
or a similar function, to regenerate the visual appearance of the field to reflect the updated value. This ensures the changes are visually apparent in the rendered PDF. Without this step, the data might be correctly stored, but the form’s visual display wouldn’t show the changes. This refresh is crucial for a seamless user experience if the filled form is to be viewed directly.
Handling Different Field Types (Text, Checkboxes, etc.)
PDF forms encompass various field types beyond simple text boxes. Checkboxes, radio buttons, dropdown lists, and others demand specific handling. For text fields, setting the value is straightforward using a setValue
-like method. Checkboxes and radio buttons often require setting a boolean value (true/false) representing checked/unchecked states. Dropdown lists might necessitate setting the selected item’s index or value. The chosen Java library will provide specific methods for each field type. Understanding these nuances is essential. Failure to account for different field types can lead to incorrect form population or even exceptions during processing. Always consult the library’s documentation for detailed instructions on handling the diverse field types present in your target PDF forms.
Working with FDF (Form Data Format)
FDF (Form Data Format) offers an efficient mechanism for exchanging form data between PDF documents and applications. It’s essentially a text-based format that describes the values of fields within a PDF form. Many Java PDF libraries support FDF import and export. To fill a form using FDF, you create an FDF file specifying field names and their corresponding values. Then, your Java code uses the library to import this FDF data into the target PDF, effectively filling the form. Similarly, you can export form data from a completed PDF to an FDF file for later use or storage. This approach is especially beneficial when dealing with a large number of forms or when automating the process of filling and saving many forms. Libraries often provide convenient methods for working directly with FDF files, streamlining the interaction between your Java code and the PDF forms.
Advanced Techniques
Explore techniques for handling complex forms, robust error handling, and optimizing performance when processing numerous or large PDF forms programmatically using Java.
Processing Complex Forms
Programmatically handling complex PDF forms in Java requires robust strategies. Nested structures, dynamic content, and intricate layouts demand careful consideration. Libraries like iText and Apache PDFBox offer tools to navigate these complexities. Understanding the form’s structure—identifying fields, their relationships, and any conditional logic—is crucial. Iterative processing, where you systematically traverse the form’s hierarchy, may be necessary. For instance, you might process fields within a particular section before moving to another. Error handling is critical to prevent unexpected crashes. Consider using try-catch blocks to gracefully manage exceptions such as missing fields or invalid data types. Regularly test your code with diverse and complex forms to ensure reliable performance and accurate data population. Remember to validate user inputs before populating the form to avoid data inconsistencies or security vulnerabilities.
Error Handling and Validation
Robust error handling is vital when programmatically filling PDF forms in Java. Unexpected issues, like missing fields or incorrect data types, can halt processing. Employ try-catch blocks to gracefully manage exceptions. Validate user inputs before populating the form to prevent data inconsistencies or security vulnerabilities. Check data types, lengths, and formats against expected field specifications. For instance, ensure numeric fields receive numbers and text fields receive strings of appropriate length. Handle exceptions specific to the chosen PDF library (e.g., IOExceptions for file access). Log errors comprehensively for debugging. Provide informative error messages to the user, guiding them towards resolving input issues. Consider implementing custom validation rules based on specific form requirements, using regular expressions or custom validation functions. Thorough testing with various input scenarios is essential to identify and address potential error conditions. This proactive approach ensures the reliability and robustness of your form-filling application.
Optimizing Performance for Large Forms
Processing extensive PDF forms programmatically in Java demands performance optimization. For large forms with numerous fields, direct field manipulation can be slow. Consider batch processing⁚ group field updates to minimize interactions with the PDF library. Employ efficient data structures to manage field data, such as HashMaps for quick field lookups. Avoid redundant operations⁚ only access and modify fields necessary. Optimize I/O operations⁚ use buffered streams for reading and writing PDF data to reduce disk access. If feasible, process the form in parts, handling sections independently to improve responsiveness. Explore advanced features of your PDF library (e.g., caching mechanisms). Profile your code to pinpoint performance bottlenecks and focus optimization efforts accordingly. Employ asynchronous processing, particularly if dealing with network-based data sources. Pre-process data to standardize it before populating the form, reducing processing time during form filling. Memory management is crucial; release resources promptly to avoid memory leaks, particularly when dealing with large files. These strategies ensure efficient handling of large forms.
Example Implementations
This section showcases practical examples using popular Java libraries like Apache PDFBox, iText, Aspose.PDF, and Spire.PDF to fill PDF forms programmatically.
Using Apache PDFBox
Apache PDFBox, a robust open-source Java library, offers comprehensive functionalities for PDF manipulation. To fill forms using PDFBox, you first load the PDF document. Then, access individual form fields by their names. Use the setValue
method to populate each field with the corresponding data. Remember to refresh the appearance stream using refreshAppearance
after modifying field values to ensure accurate visual representation of the changes within the PDF form. Error handling is crucial; anticipate potential exceptions like IOException
during file access and handle them gracefully to prevent application crashes. This ensures the robustness of your PDF form filling process. For complex forms, iterative processing of fields might be necessary. Efficiently managing resources, like closing the PDF document after processing, is essential for optimal performance, especially when dealing with numerous files or large documents. The open-source nature of PDFBox contributes to its widespread adoption and community support.
Using iText
iText is a powerful commercial Java library for PDF manipulation, including form filling. Begin by creating a PdfReader
instance to open your PDF form. Next, use a PdfStamper
to add content without modifying the original. Access form fields using their names via getField
. Set field values with the setValue
method, handling different field types (text, checkboxes, etc.) appropriately. Remember to call setFullCompression
for efficient file size management. iText’s robust error handling helps manage exceptions during the process, such as IOExceptions
. For optimal performance, especially with large forms, consider using techniques like batch processing or asynchronous operations. iText’s comprehensive documentation and community support provide valuable resources for troubleshooting and advanced usage. Its commercial license offers dedicated support and ensures access to regular updates and bug fixes.
Using Aspose.PDF for Java
Aspose.PDF for Java provides a comprehensive API for working with PDF documents, including form filling. Start by loading your PDF form using Document doc = new Document(src);
. Access individual form fields by name using the getFields
method. Populate these fields using field.setValue("yourValue");
, handling text fields, checkboxes, and other types as needed. Aspose.PDF’s robust features ensure handling of complex form structures, including nested fields and conditional logic. For enhanced efficiency, consider batch processing or asynchronous operations when dealing with large forms. Error handling is simplified through Aspose’s clear exception management system, making debugging easier. This library’s functionality extends beyond simple form filling, offering features for creating, merging, and manipulating PDFs. Aspose.PDF’s active community support and detailed documentation provide valuable assistance during development.
Using Spire.PDF for Java
Spire.PDF for Java offers a straightforward approach to programmatically filling PDF forms. Begin by loading the PDF using PdfDocument doc = new PdfDocument.LoadFromFile("path/to/your/form.pdf");
. Access form fields via their names using PdfFormFields fields = doc.Form;
followed by PdfFormField field = fields.GetField("fieldName");
. Set values using field.Value = "yourValue";
for various field types. Spire.PDF simplifies handling of different field types like text fields, checkboxes, and radio buttons. Its intuitive API simplifies the process. For complex forms, consider using iterators to efficiently process numerous fields. Error handling is integrated into the library, providing informative exceptions for easier debugging. After filling, save the modified PDF using doc.SaveToFile("path/to/filled/form.pdf");
. Spire.PDF’s comprehensive documentation and examples facilitate quick integration and efficient form population.
Best Practices and Considerations
Prioritize data security and protection when handling sensitive information within PDF forms. Implement robust error handling and validation to ensure data integrity and prevent unexpected issues.
Security and Data Protection
When programmatically filling PDF forms in Java, robust security measures are crucial, especially when dealing with sensitive data. Employ encryption techniques to protect data both in transit and at rest. Validate all user inputs to prevent injection attacks and ensure data integrity. Utilize trusted libraries and regularly update them to patch vulnerabilities. Consider implementing access control mechanisms to restrict who can access and modify the forms. If storing filled forms, use secure storage solutions with appropriate access controls. Regularly audit your code and processes to identify and address potential security weaknesses. Remember that neglecting security best practices can lead to data breaches and compromise sensitive information. Always adhere to relevant data privacy regulations and industry standards. Thorough testing and validation are also essential to ensure your implementation is secure and reliable. Implement logging and monitoring to detect and respond to suspicious activity promptly.
death on the nile pdf
You May Also Like
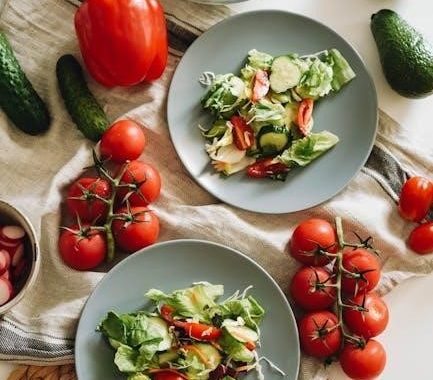
carnivore diet food list pdf
June 11, 2025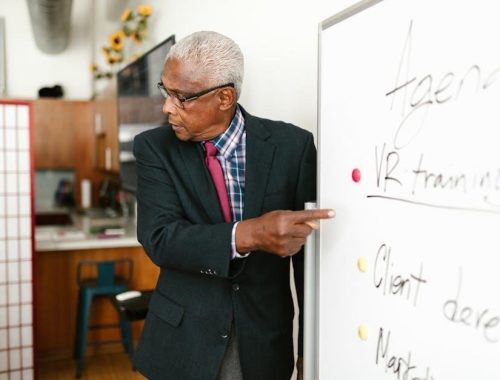
instructions for tie dye washing
June 4, 2025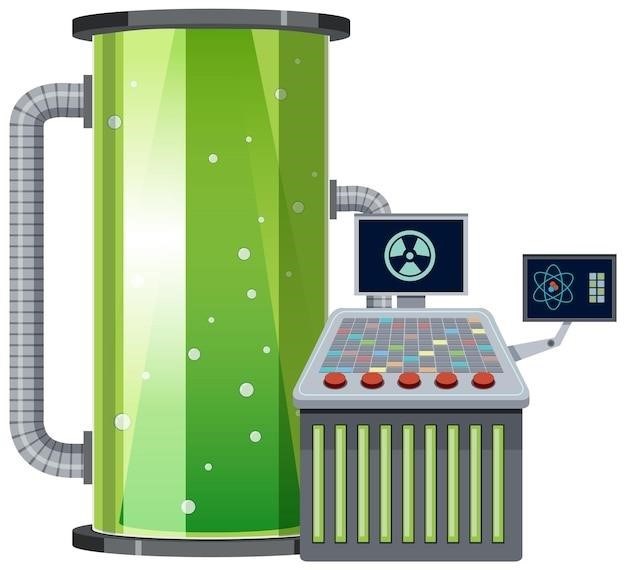